Elixir Process
Elixir Process[edit]
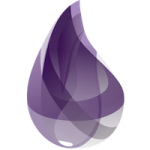
The Elixir programming language provides a powerful and concurrent programming model through its process-oriented architecture. In Elixir, processes are lightweight and isolated units of execution that communicate with each other through message passing. This article explores the concept of processes in Elixir and explains how they can be used to build highly reliable and scalable applications.
Introduction to Elixir Processes[edit]
Elixir processes are not operating system processes but rather abstractions provided by the Elixir runtime. They are lightweight and can be spawned, managed, and supervised efficiently. Each Elixir process has its own memory space, garbage collector, and execution context, making them ideal for building concurrent and fault-tolerant systems.
Spawning Processes[edit]
In Elixir, processes can be spawned using the `spawn/1` function, which takes a function as an argument and executes it in a separate process. The spawned process runs concurrently with the calling process and can communicate with other processes through message passing.
pid = spawn(fn -> IO.puts "Hello, Elixir process!" end)
Message Passing[edit]
Message passing is a key mechanism for inter-process communication in Elixir. Processes can send messages to each other using the `send/2` function and receive messages using the `receive/1` macro. Messages are asynchronous, meaning that the sender does not block waiting for a response.
send(pid, {:message, "Hello"})
receive do {:message, content} -> IO.puts content end
Process Monitoring[edit]
Elixir provides a powerful process monitoring mechanism through the `Process.monitor/1` and `Process.monitor/2` functions. Monitoring allows processes to be notified when another process crashes or terminates. This feature is crucial for building fault-tolerant systems.
ref = Process.monitor(pid)
receive do {:DOWN, ref, :process, pid, reason} -> IO.puts "Process terminated: #{reason}" end
GenServer[edit]
`GenServer` is a behavior module in Elixir that simplifies the implementation of server processes. It provides a standardized API for handling messages, state management, and error handling. GenServers are widely used in Elixir applications to encapsulate business logic and manage state.
defmodule MyServer do use GenServer def start_link do GenServer.start_link(__MODULE__, []) end def init(_) do {:ok, []} end def handle_info(:ping, state) do {:noreply, state} end end
Supervision[edit]
Supervision is a powerful concept in Elixir that allows building fault-tolerant systems. With supervision, processes can be organized into supervision trees, where each process is supervised by another process. If a supervised process crashes, the supervisor can take appropriate actions such as restarting the process. This ensures the system remains resilient and operational even in the face of failures.
defmodule MyApp.Supervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init(_) do children = [ worker(MyServer, []) ] supervise(children, strategy: :one_for_one) end end
Conclusion[edit]
Elixir processes provide a robust and scalable foundation for building concurrent applications. With features like lightweight spawning, message passing, process monitoring, and supervision, Elixir enables developers to create fault-tolerant systems that can handle complex concurrent tasks. Understanding the concepts and practices around Elixir processes is essential for harnessing the full potential of the Elixir programming language.