GenServer in Elixir
GenServer in Elixir[edit]
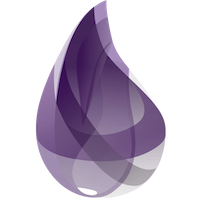
GenServer is a fundamental behavior in the Elixir programming language. It is a part of the `:gen` module, which provides a generic server implementation for building concurrent and fault-tolerant systems. GenServer can be used to create processes that encapsulate state and define a set of callbacks to handle incoming messages.
Features[edit]
Some key features of GenServer are:
- **Concurrency**: GenServer allows developers to create multiple server processes that can run concurrently, enabling parallel execution.
- **State Management**: Each GenServer process has its own isolated state, allowing developers to keep track of information specific to that process.
- **Asynchronous Messaging**: GenServer provides the ability to send and receive messages between processes, making it easy to communicate and share information.
- **Fault Tolerance**: GenServer comes with built-in mechanisms for handling errors and failures, ensuring that systems can recover from crashes and continue running reliably.
Callbacks[edit]
When defining a GenServer, several callbacks need to be implemented:
- **`init/1`**: Initializes the server state and returns it.
- **`handle_call/3`**: Handles synchronous messages, also known as "calls", received by the server.
- **`handle_cast/2`**: Handles asynchronous messages, also known as "casts", received by the server.
- **`handle_info/2`**: Handles non-call or non-cast messages received by the server.
- **`terminate/2`**: Cleans up the server state before it terminates.
Usage[edit]
To use GenServer, follow these steps:
1. Define a module and use the `GenServer` behavior.
2. Implement the required callbacks.
3. Start the GenServer using `GenServer.start/3` or `GenServer.start_link/3`.
4. Communicate with the GenServer using `GenServer.call/2`, `GenServer.cast/2`, or other related functions.
An example usage looks like this:
```elixir defmodule MyServer do
use GenServer
def init(initial_state) do {:ok, initial_state} end
def handle_call(:get_state, _from, state) do {:reply, state, state} end
def handle_cast({:set_state, new_state}, state) do {:noreply, new_state} end
end
{:ok, pid} = GenServer.start(MyServer, initial_state) GenServer.call(pid, :get_state) #=> the initial_state GenServer.cast(pid, {:set_state, :new_state}) ```
Conclusion[edit]
GenServer is a powerful tool in Elixir for building concurrent and fault-tolerant systems. With its rich set of features and intuitive API, developers can create robust applications that leverage the benefits of process-based concurrency. Understanding how to use GenServer effectively is essential for mastering Elixir programming.