Process Monitoring in Elixir
Process Monitoring in Elixir[edit]
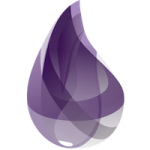
Process Monitoring in Elixir is a vital aspect of building resilient and fault-tolerant systems. Elixir, a functional programming language built on the Erlang Virtual Machine (BEAM), provides powerful tools and mechanisms for monitoring and managing concurrent processes.
The Process Model in Elixir[edit]
Elixir follows the actor model, where concurrent processes are the primary abstraction for building scalable and fault-tolerant applications. Each process runs independently and communicates with other processes through message passing.
In Elixir, processes are lightweight and have a small memory footprint, allowing for the creation of thousands or even millions of concurrent processes. Each process is identified by a unique process identifier (PID), which is used to send messages and interact with the process.
Basic Process Monitoring[edit]
Elixir provides several built-in functions and mechanisms for basic process monitoring. These include:
- `spawn/1` and `spawn/3`: Functions used to create new processes and execute code in parallel.
- `send/2` and `receive/1`: Functions used for message passing between processes.
- `self/0` and `pid/3`: Functions to retrieve the PID of the current process or convert a PID from a reference.
- `link/1` and `unlink/1`: Functions used to establish and break links between processes.
- `Process.alive?/1`: Function to check if a process is alive.
- `Process.exit/2`: Function used to terminate a process and define an exit reason.
OTP Supervision Trees[edit]
In Elixir, OTP (Open Telecom Platform) provides a set of battle-tested abstractions for building fault-tolerant systems. OTP introduces the concept of supervision trees, which allow for hierarchical process supervision and fault recovery.
A supervision tree is a hierarchical structure, where one or more supervisor processes monitor and control a group of worker processes. If a worker process crashes, the supervisor can decide how to handle the failure, whether it's restarting the process, terminating it permanently, or even stopping the whole tree.
The `Supervisor` module in the `:supervisor` OTP behavior is used to define and manage supervision trees. It provides a set of callbacks and strategies for handling failures and restarting processes.
Process Monitoring with `:observer`[edit]
Elixir comes bundled with a powerful tool called `:observer`, which provides a graphical interface for monitoring and inspecting the state of the BEAM runtime system. The `:observer` module allows developers to visualize processes, applications, ports, and other system resources, making it easier to understand and debug complex distributed systems.
To start the observer, simply run the following code in Elixir's interactive shell:
```elixir
- observer.start()
```
The `:observer` window will open, providing a wealth of information and tools for process monitoring and system analysis.
Conclusion[edit]
Process monitoring is a critical aspect of building resilient and fault-tolerant systems in Elixir. With its lightweight processes, built-in mechanisms, OTP supervision trees, and tools like `:observer`, Elixir empowers developers to create highly concurrent and reliable applications.